Last Updated on :
Friday, 28 January 2022
Mega Menu in WIX Website
Pack your entire website's navigation into a single multi-level dropdown menu.
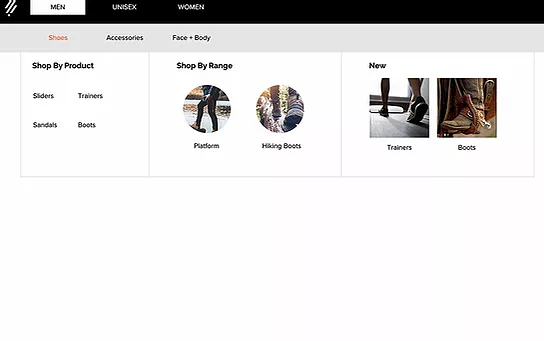
In this Tutorial, we create a dropdown menu that allows your site's visitors to easily navigate through the different categories of your site. For example, under the MEN category, there is a subcategory, SHOES. In that subcategory visitors can filter by Product, Range and New.
View Example Site ➡ || Edit Example Site ➡
Code used to make mega Menu
import wixData from 'wix-data';
import wixLocation from 'wix-location';
$w.onReady(function () {
let allMenusData;
let $item = $w.at();
let containers = $item("Container");
iniPage()
.then((resuts) => {
allMenusData = resuts;
selectMenu(allMenusData, 'subMenuRepeater', 0);
let mainMenuRepeaterData = $w("#mainMenuRepeater").data;
let subMenuRepeaterData = $w("#subMenuRepeater").data;
$w("#mainMenuRepeater").forItems([mainMenuRepeaterData[0]._id], ($item, itemData, index) => {
$item('#mainMenuButton').style.backgroundColor = 'white';
$item('#mainMenuButton').style.color = 'black';
$item('#subMenuButton').style.color = 'black';
});
$w("#subMenuRepeater").forItems([subMenuRepeaterData[0]._id], ($item, itemData, index) => {
$item('#subMenuButton').style.color = '#ED5829';
});
});
containers.forEach(function (container, i, repeatersArray) {
const repeater = container.parent;
const containerChildern = container.children;
const image = containerChildern.filter(element => element.type === '$w.Image')[0];
const button = containerChildern.filter(element => element.type === '$w.Button')[0];
repeater.onItemReady(($item, itemData, index) => {
$item('#' + button.id).label = itemData.name;
if (itemData.image) {
$item('#' + image.id).src = itemData.image;
}
if (itemData.link) {
$item('#' + container.id).onClick((event) => {
// if you the menu to link to different pages, add the needed links to the collections and uncomment the following line:
// wixLocation.to(itemData.link);
});
} else {
$item('#' + button.id).onMouseIn((event) => {
switch (button.id) {
case 'mainMenuButton':
button.style.backgroundColor = 'black';
$item('#' + button.id).style.backgroundColor = 'white';
$item('#' + button.id).style.color = 'black';
break;
case 'subMenuButton':
button.style.color = 'black';
$item('#' + button.id).style.color = '#ED5829';
break;
default:
break;
}
selectMenu(allMenusData, repeater.id, index);
});
}
});
});
});
async function iniPage() {
try {
const allMainMenuData = await wixData.query('mainMenu').limit(1000).find();
$w('#mainMenuRepeater').data = allMainMenuData.items;
$w('#mainMenuStrip').expand();
const allSubMenuData = await wixData.query('subMenu').limit(1000).find();
$w('#subMenuRepeater').data = allSubMenuData.items.filter(item => item.mainMenu === allMainMenuData.items[0]._id);
$w('#subMenuStrip').expand();
const allRangeData = await wixData.query('range').limit(1000).find();
const allNewData = await wixData.query('new').limit(1000).find();
const allProductData = await wixData.query('product').limit(1000).find();
return {
'mainMenuData': allMainMenuData.items,
'subMenuData': allSubMenuData.items,
'rangeData': allRangeData.items,
'newData': allNewData.items,
'productData': allProductData.items
};
} catch (err) {
console.log('error ', err);
}
}
function selectMenu(allMenusData, clickedMenu, clickedButton) {
const allMainMenuData = allMenusData.mainMenuData;
switch (clickedMenu) {
case 'mainMenuRepeater':
$w('#subMenuRepeater').data = allMenusData.subMenuData.filter(item => item.mainMenu === allMainMenuData[clickedButton]._id);
$w('#megaMenuStrip').collapse();
break;
case 'subMenuRepeater':
$w('#rangeRepeater').data = filterd(allMenusData.rangeData);
$w('#newRepeater').data = filterd(allMenusData.newData);
$w('#productRepeater').data = filterd(allMenusData.productData);
$w('#megaMenuStrip').expand();
break;
default:
break;
}
function filterd(data) {
return data.filter(item => item.subMenu === ($w('#subMenuRepeater').data)[clickedButton]._id);
}
}
How We Built It
Collections For this example, we created five collections:
mainMenu - Contains the names that show on each main menu button label.
subMenu - Contains the names that show on each submenu button label. Each submenu item references which main menu item it belongs under.
product - Contains information about products. Each item references the submenu item it belongs under
new - Same as the Product collection, but for new products.
range - Same as the Product collection, but for product ranges.
Page Elements In our site we added the following repeaters that make up the menu:
mainMenuRepeater - for the main menu buttons.
subMenuRepeater - for the submenu buttons.
productRepeater - for displaying the products that belong to a submenu item.
rangeRepeater - for displaying the product ranges that belong to a submenu item.
newRepeater - for displaying the new products that belong to a submenu item
Code In this example we added the following code to create the mega menu:
In $w.onReady:
Call the iniPage function to query all the menu data and show the main and sub menus.
Use containers.forEach to loop over all the repeater containers and implement the functionality of the repeaters contents, such as populating images and setting button link.
Note: In this example, we defined a generic link to 'wix.com', change it to your own link.
The selectMenu function:
Defines the different states of the buttons and the data of the repeaters depending on what menu item was clicked.
Returns data that is filtered to only include information that relates to the current selection.